AppleButton + -> AppletButton + <-
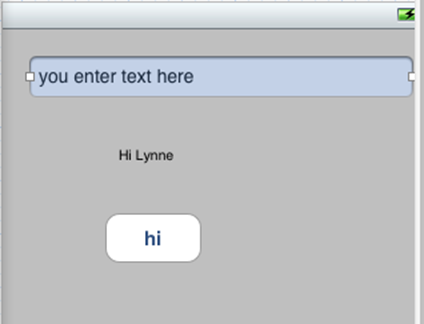
//
// HITest_ViewController.h
// HiTest
//
// Created by student on 12/4/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface HITest_ViewController : UIViewController <UITextFieldDelegate> {
NSString *currentMessage;
IBOutlet UITextField *textField;
IBOutlet UILabel *messageField;
IBOutlet UIButton *change_message_button;
}
- (IBAction)showMessage:(id)sender;
//new method to call when Return button hit on TextField this class serves as delegate for
- (BOOL)textFieldShouldReturn:(UITextField *)tf;
@end
//
// HITest_ViewController.m
// HiTest
//
// Created by student on 12/4/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "HITest_ViewController.h"
@interface HITest_ViewController ()
@end
@implementation HITest_ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
}
- (void)viewDidUnload
{
textField = nil;
[super viewDidUnload];
// Release any retained subviews of the main view.
}
- (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation
{
return (interfaceOrientation != UIInterfaceOrientationPortraitUpsideDown);
}
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if(self) {
//setup default message
currentMessage = @"Hi 4521";
}
return self;
}
//method to handle event when button hit
- (IBAction)showMessage:(id)sender
{
//get current typed in message
currentMessage = [textField text];
NSLog(@"going to display message %@", currentMessage);
//display new meeage
[messageField setText:currentMessage];
}
//method to get rid of keyboard
- (BOOL)textFieldShouldReturn:(UITextField *)tf
{
[tf resignFirstResponder];
NSLog(@"Entering textFieldShouldReturn");
return YES;
}
@end
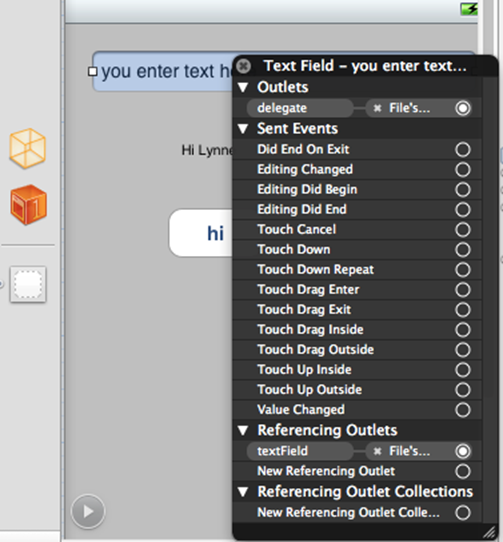