Android Intents
- an abstract description of an operation to be performed
- are system (asynchronous) messages, running around the inside of the device, notifying applications of various events
- Intents are a powerful concept as they allow to create loosely coupled applications
- Intents can be used to go from one Activity to another Activity ---we will concentrate on this initially and the examples in this webpage show such examples!!!
- hardware state changes (e.g., an SD card was inserted)
- incoming data (e.g., an SMS message arrived)
- application events (e.g., your activity was launched from the device's main menu)
- request to run a built-in application (e.g. browser, phone, map)
- application could ask via an intent for a contact application
An application can call directly a service or activity .
An application can ask the Android system for registered services and applications for an intent
- In Manifest File, each activity can be declared to have one or more <intent-filter> tags.
- <intent-filter> defines how another Activity can invoke this activity through the creation of an Intent with it (we will see examples below).
- You MUST do this!!
The category of android.intent.category.DEFAULT means any activity can invoke this MySecondActivity activity through an Intent. (we will see how this is done next). This means that we can create this activity with a call to a methods....we will see how to do this in the next section.
You may recall up to now, many of our previous projects had the starting activity that was launched with the application and the category was then defined as android.intent.category.LAUNCHER
AndroidManifest.xml
<xml ************
<activity android:name=".MySecondActivity" android:label="MySecondActivity" >
<intent-filter>
<action android:name="grewe.mylab.SecondActivity" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
</activity>
***************
REST of this Page concentrates on Intents and using them to start Activities
Android Intents to call another Activity (from book)
Project is called UsingIntents Has 2 Activities
ACTIVITY 1 UsingIntentsActivity (started at launch of application ---see AndroidManifest.xml below) whose interface (main.xml) has a Button
"Display second activity"
-
in AndroidManifest.xml has <intent-filter> that launches this at launch of application
-
has button with onClick(*) will create a new Intent to "start" the SecondActivity using startActivity(*) method
ACTIVITY 2 SecondActivity simply has UI to ask user for their name (we will expand in next example)
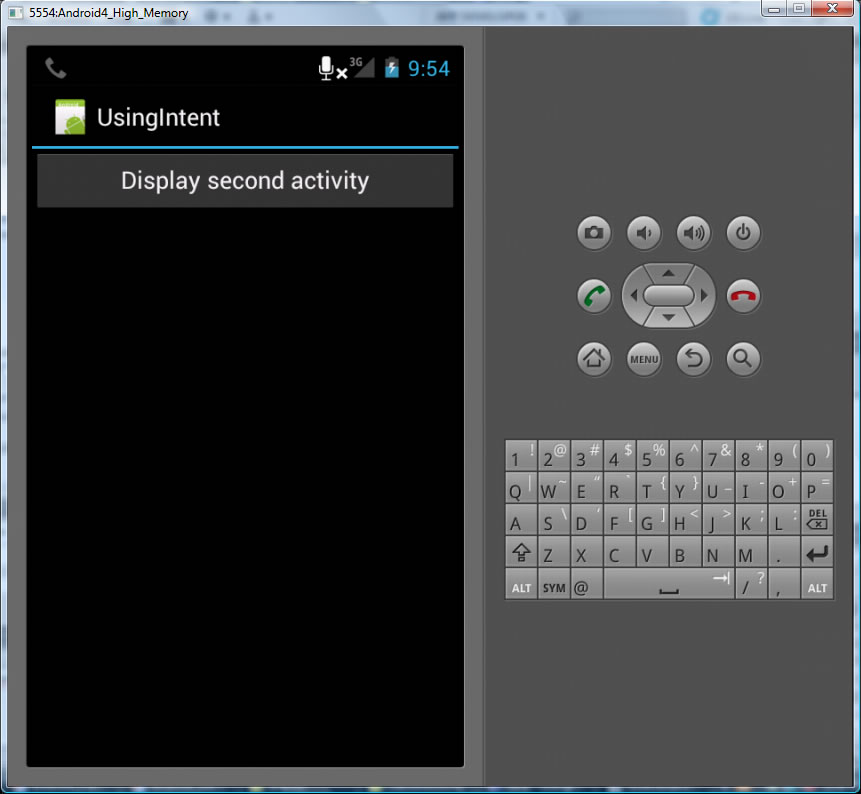
|
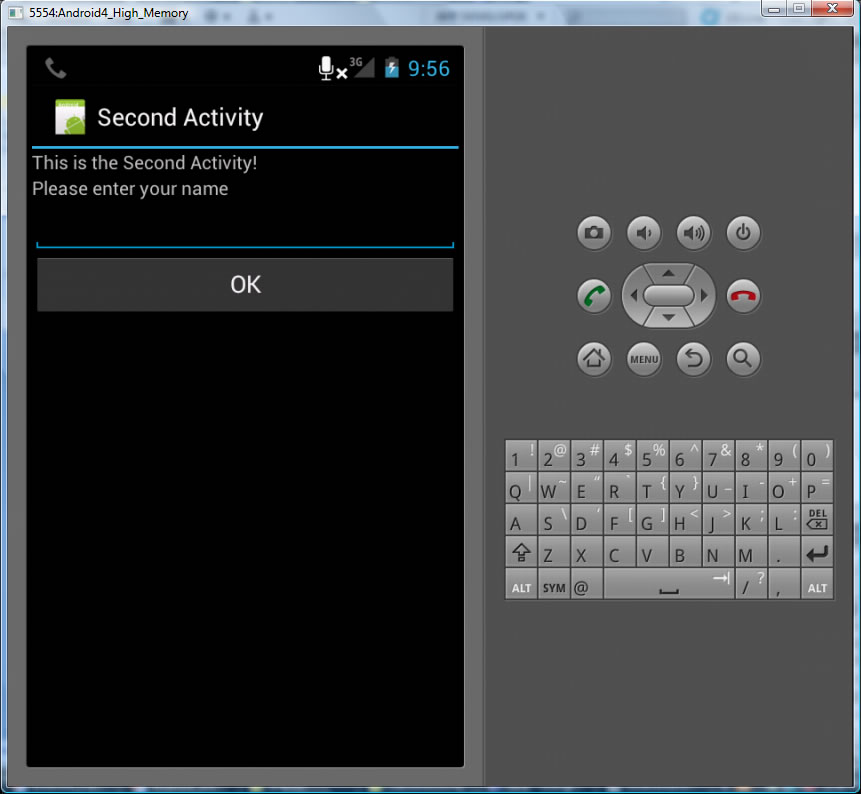
|
AndroidManifest.xml - has 2 Activities
UsingIntentActivity (is launched at launch time)
SecondActivity (is setup so )
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="net.learn2develop.UsingIntent"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk android:minSdkVersion="14" />
<application
android:icon="@drawable/ic_launcher"
android:label="@string/app_name" >
<activity
android:label="@string/app_name"
android:name=".UsingIntentActivity" >
<intent-filter >
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity
android:label="Second Activity"
android:name=".SecondActivity" >
<intent-filter >
<action android:name="net.learn2develop.SecondActivity" />
</intent-filter>
</activity>
</application>
</manifest> |
main.xml (interface for UsingInentActivity --our launch activity)
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Display second activity"
android:onClick="onClick"/>
</LinearLayout> |
UsingIntentActivity.java (our launch activity) --when button clicks starts SecondActivity
package net.learn2develop.UsingIntent;
import android.app.Activity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.view.View;
import android.widget.Toast;
public class UsingIntentActivity extends Activity {
int request_Code = 1;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
//start the SecondActivity Activity by using startActivity(*) method // passing to it a new Intent object based on SeconActivity
public void onClick(View view) {
startActivity(new Intent("net.learn2develop.SecondActivity"));
}
} |
secondactivity.xml -- GUI for SecondActivity with edit text area for name and dummy button
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="This is the Second Activity!" />
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Please enter your name" />
<EditText
android:id="@+id/txt_username"
android:layout_width="fill_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btn_OK"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="OK"
android:onClick="onClick"/>
</LinearLayout>
|
SecondActivity.java -- just puts up its xml defined GUI on creation
package net.learn2develop.UsingIntent;
import android.app.Activity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
public class SecondActivity extends Activity{
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.secondactivity);
}
}
|
More on How to Create Intents
OPTION 1:Simple Intent i = new Intent(action_statetment);
= represents either a built-in application or the name of an activity specified in your AndroidManifest.xml file
Intent i = new Intent(android.content.Intent.ACTION_VIEW); ///specifies the viewer (web browser) built-in app
Intent i = new Intent(android.content.Intent.ACTION_DIAL); //specifies phone built-in app
- android.content.Intent.ACTION_VIEW //view some data passed
- android.content.Intent.ACTION_DIAL //show phone dialer
- android.content.Intent.EDIT //edit data passed
- android.content.Intent.ACTION_PICK //contacts database
See above example new Intent("net.learn2develop.SecondActivity")
Intent i = new Intent(android.content.Intent.ACTION_VIEW, Uri.parse("http://www.amazon.com")); //here parse URL want to view
startActivity(i);
Intent i = new Intent("android.content.Intent.ACTION_VIEW");
i.setData(URI.parse("http://www.amazon.com"));
Other examples
- Intent i = new Intent(android.content.Intent.ACTION_DIAL, Uri.parse("tel:+5101234567"));
- Intent i = new Intent(android.content.Intent.ACTION_CALL, Uri.parse("tel:+5101234567")); //if add permission to application manifest "android.permission.CALL_PHONE" will dial automatically without user prompting.
- Intent i = new Intent(android.content.Intent.ACTION_VIEW, Uri.parse("geo:37.8270,-122.48")); //lat and long of map
Intent i = new Intent(android.content.Intent.ACTION_PICK);
i.setType(android.provider.ContractsContract.Contracts.CONTENT_TYPE); //MIME type informaiton...content from the Contracts application
Returning Results from Intent --extending previous example
After Typing in "Lynne Grewe" in SecondActivity
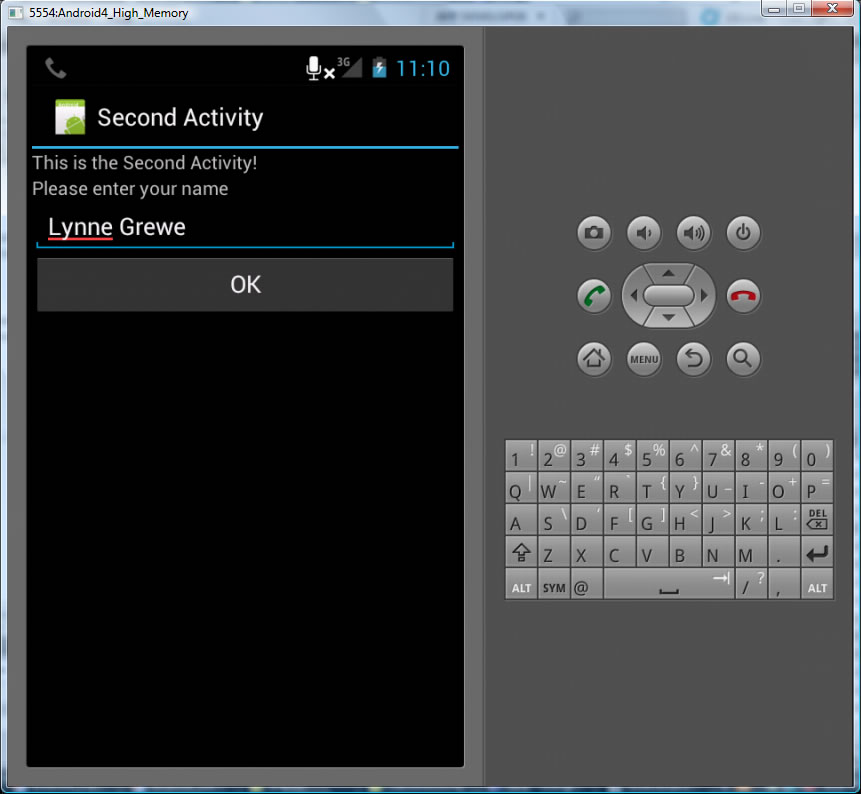
|
Inside UsingIntentActivity after getting results of Entering Name in SecondActivity
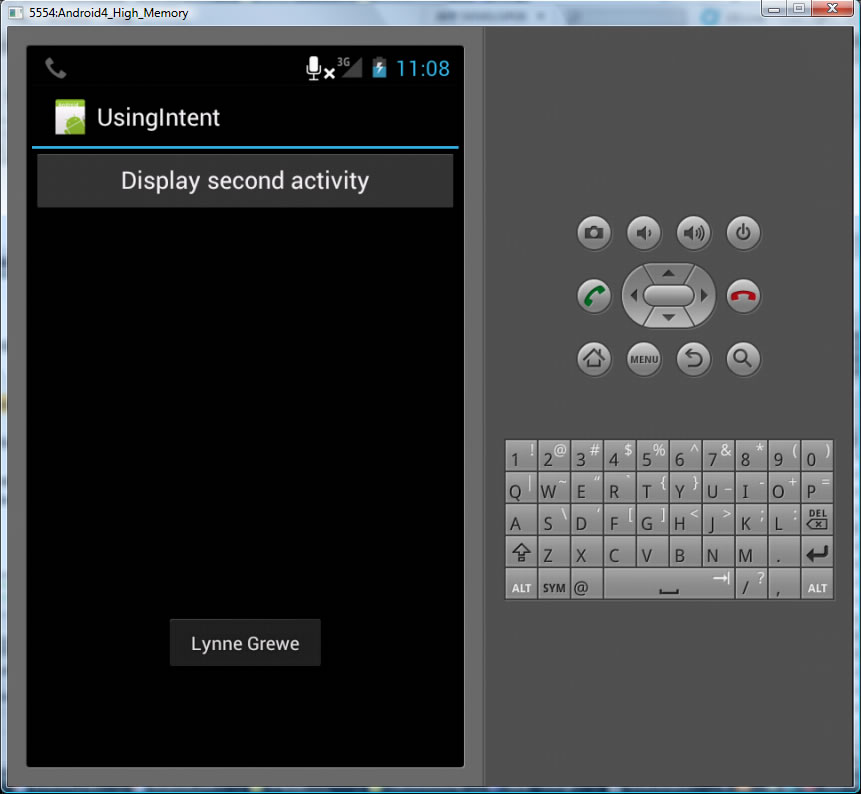
|
NOW when user types in their name in SecondActivity and hits OK you are to return results to the first Activity, UsingIntentActivity.
- STEP 1: To do this you start the second activity with the startActivityForResults(*)
- STEP 2: Inside the SecondActivity you must now do something when the button is hit and return results method.
- STEP 3: Finally the first Activity that can get the results, UsingIntentAcitivity, must implement the callback funciton onActivityRestult(*)
- In example below this method will show the name the user typed at the bottom of the UsingIntentActivity window.
- You get the set intent_object from STEP2
- OPTION 1: if data was associated with this intent with setData() use getData() to retrieve it
- OPTION 2: if data was associated with this intent with putExtra("name", value) use get*Extra("name", default_value) where * is the data type of value
- OPTION 3: if data was associated with this intent with a bundle and putExtras(bundle), then do Bundle bb = Intent_returned.getExtras(), bb.get*("name");
public void onActivityResult(int requestCode, int resultCode, Intent data)
{
if (requestCode == request_Code) {
if (resultCode == RESULT_OK) {
Toast.makeText(this,data.getData().toString(), Toast.LENGTH_SHORT).show();
Toast.makeText(this,data.getIntExtra("age3", -1),, Toast.LENGTH_LONG).show(); //using getIntExtra as value pu was int
}
}
}
NOTE: Ignore the ThirdActivity ---used in next section of this webpage.
AndroidManifest.xml -- NOTHING CHANGED REALLY
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="net.learn2develop.UsingIntent"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk android:minSdkVersion="14" />
<application
android:icon="@drawable/ic_launcher"
android:label="@string/app_name" >
<activity
android:label="@string/app_name"
android:name=".UsingIntentActivity" >
<intent-filter >
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity
android:label="Second Activity"
android:name=".SecondActivity" >
<intent-filter >
<action android:name="net.learn2develop.SecondActivity" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
</activity>
<activity
android:label="Third Activity"
android:name=".ThirdActivity" >
<intent-filter >
<action android:name="net.learn2develop.SecondActivity" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
</activity>
</application>
</manifest> |
main.xml ---NOTHING CHANGED
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Display second activity"
android:onClick="onClick"/>
</LinearLayout> |
UsingIntentActivity.java --- SEE CHANGES
package net.learn2develop.UsingIntent;
import android.app.Activity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.view.View;
import android.widget.Toast;
public class UsingIntentActivity extends Activity {
int request_Code = 1;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
//create SecondActivity.
public void onClick(View view) {
startActivityForResult(new Intent("net.learn2develop.SecondActivity"), request_Code);
}
//callback function to get results from SecondActivity when it is done
public void onActivityResult(int requestCode, int resultCode, Intent data)
{
if (requestCode == request_Code) {
if (resultCode == RESULT_OK) {
Toast.makeText(this,data.getData().toString(),
Toast.LENGTH_SHORT).show();
}
}
}
} |
seconactivity.xml ---NOTHING CHANGED
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="This is the Second Activity!" />
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Please enter your name" />
<EditText
android:id="@+id/txt_username"
android:layout_width="fill_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btn_OK"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="OK"
android:onClick="onClick"/>
</LinearLayout>
|
SecondActivity.java --when its button hit get text typed in and return results to first activity that created it
package net.learn2develop.UsingIntent;
import android.app.Activity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
public class SecondActivity extends Activity{
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.secondactivity);
}
//method to return results when Button in SecondActivity is clicked
public void onClick(View view) {
Intent data = new Intent();
//---get the EditText view---
EditText txt_username =
(EditText) findViewById(R.id.txt_username);
//---set the data to pass back---
data.setData(Uri.parse(
txt_username.getText().toString()));
//---closes the activity---
finish(); //<<<this CLOSES THIS ACTIVITY
}
}
|
Passing Data to an Activity using an Intent --extending previous example
In this simple example from book we are going to play with passing data from one Activity to Another via an Intent that starts the second activity. Go to book source code Book/Android/Source Code/PassingData
- PASS DATA TO SECOND ACTIVITY DURING ITS START: To do this you start the second activity with the startActivityForResults(i,*) where i = Intent Object that has data we are passing !!! Notice that you will pass various string values associated with the names "str1", "age1", "str2", "age2". These name value pairs are stored in an instance of a class called android.os.Bundle that is stored in the Intent i using the i.putExtras(bundle) method.
- STEP 1: Create intent for second activity (Intent i= new Intent ("*****SecondActivity); )
- STEP 2: Create instance of android.os.Bundle called extras
- STEP 3: For each (Name, Value) pair of data you want to pass, store inside Bundle using extras.putDATATYPE("name",value). Example of this is extras.putInt("age1", 25) and extras.putString("str1", "This is a string");
- STEP 4: Attach the Bundle (extras) to the Intent for the second activity. (i.putExtras(extras); )
- STEP 5: start second activity startActivityForResult(i,1);
- GET DATA PASSED TO SECOND ACTIVITY AT ITS CREATION TIME: Inside the SecondActivity the method calls the method to get the string associated with "str1" that was passed in the Intent that invoked this Activity.
AFTER Launch of application PassingDataActrivity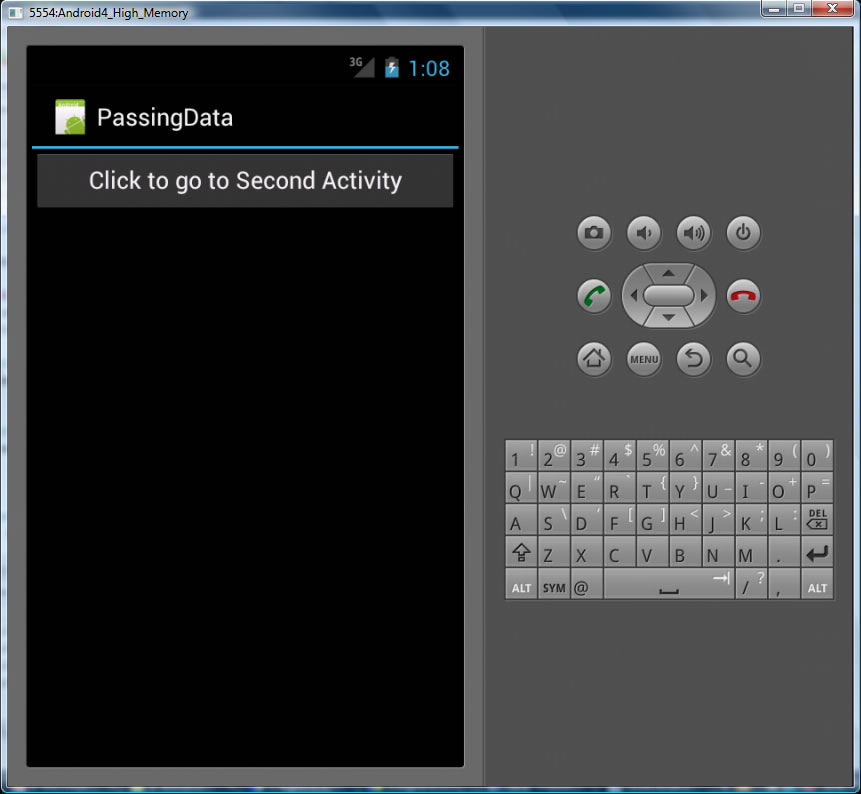 |
After going to SecondActivity-- Showing PASSED DATA --"This is a string" associated with the name "str1"--see code below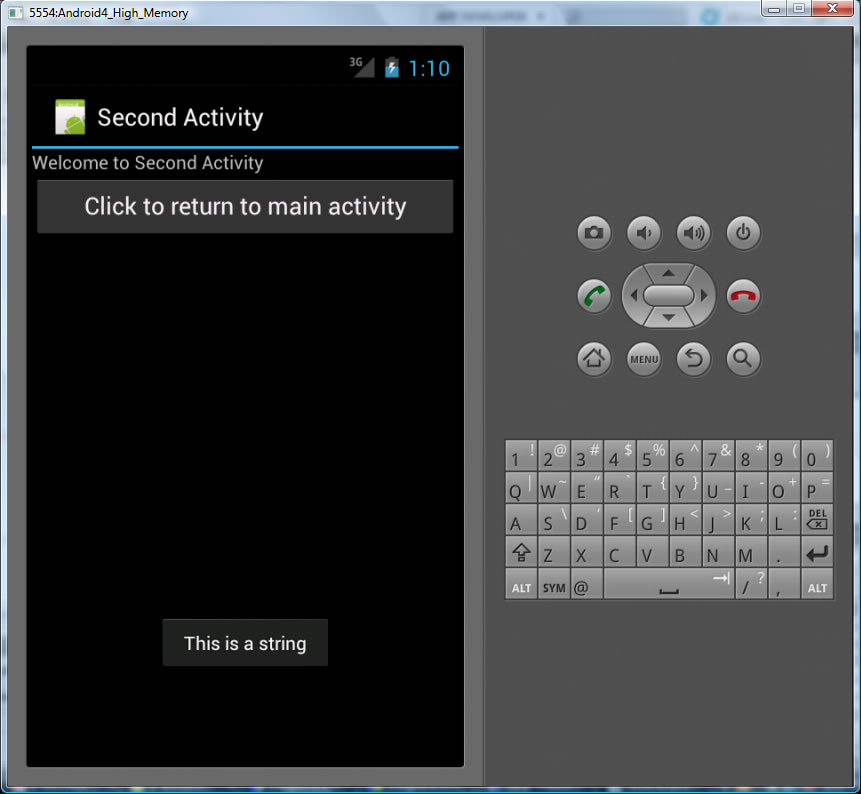 |
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="net.learn2develop.PassingData"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk android:minSdkVersion="14" />
<application
android:icon="@drawable/ic_launcher"
android:label="@string/app_name" >
<activity
android:label="@string/app_name"
android:name=".PassingDataActivity" >
<intent-filter >
<action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity
android:label="Second Activity"
android:name=".SecondActivity" >
<intent-filter >
<action android:name="net.learn2develop.PassingDataSecondActivity" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
</activity>
</application>
</manifest>
|
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<Button
android:id="@+id/btn_SecondActivity"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Click to go to Second Activity"
android:onClick="onClick"/>
</LinearLayout>
|
PassingDataActivity.java --this is the first Activity launched when Application is launched, will pass data to second activity it starts SecondActivity
package net.learn2develop.PassingData;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Toast;
public class PassingDataActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
public void onClick(View view) {
Intent i = new
Intent("net.learn2develop.PassingDataSecondActivity");
//---use putExtra() to add new key/value pairs---
i.putExtra("str1", "This is a string"); //this is what we see in screen shot above
i.putExtra("age1", 25);
//---use a Bundle object to add new key/values
// pairs---
Bundle extras = new Bundle();
extras.putString("str2", "This is another string");
extras.putInt("age2", 35);
//---attach the Bundle object to the Intent object---
i.putExtras(extras);
//---start the activity to get a result back---
startActivityForResult(i, 1);
}
public void onActivityResult(int requestCode,
int resultCode, Intent data)
{
//---check if the request code is 1---
if (requestCode == 1) {
//---if the result is OK---
if (resultCode == RESULT_OK) {
//---get the result using getIntExtra()---
Toast.makeText(this, Integer.toString(
data.getIntExtra("age3", 0)),
Toast.LENGTH_SHORT).show();
//---get the result using getData()---
Toast.makeText(this, data.getData().toString(),
Toast.LENGTH_SHORT).show();
}
}
}
} |
secondactivity.xml -
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Welcome to Second Activity" />
<Button
android:id="@+id/btn_MainActivity"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Click to return to main activity"
android:onClick="onClick"/>
</LinearLayout> |
SecondActivity.java
---note below the where we retrieve data passed to us as bundle in Intent that started this Activity.
package net.learn2develop.PassingData;
import android.app.Activity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.view.View;
import android.widget.Toast;
public class SecondActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.secondactivity);
//---get the data passed in using getStringExtra()---what we see in screen shot above
Toast.makeText(this,, Toast.LENGTH_SHORT).show();
//---get the data passed in using getIntExtra()---
Toast.makeText(this,Integer.toString( , Toast.LENGTH_SHORT).show();
//---get the Bundle object passed in---
Bundle bundle =
//---get the data using the getString()---
Toast.makeText(this, , Toast.LENGTH_SHORT).show();
//---get the data using the getInt() method---
Toast.makeText(this,Integer.toString(,Toast.LENGTH_SHORT).show();
}
public void onClick(View view) {
//---use an Intent object to return data---
Intent i = new Intent();
//---use the putExtra() method to return some
// value---
i.putExtra("age3", 45);
//---use the setData() method to return some value---
i.setata(Uri.parse("Something passed back to main activity"));
//---set the result with OK and the Intent object---
setResult(RESULT_OK, i);
//---destroy the current activity---
finish();
}
} |
One More THING --- Intent Resolution
When you have an Intent and you say you want a built-in application it will look for any Activity that is available that has this feature...and if you use Intent.createChooser(i, "string") it will give the user all the available ptions to select.
Intent i = new Intent(android.content.Intent.ACTION_VIEW, Uri.parse("http://www.amazon.com"));
startActivity(Intent.createChooser(i, "Complete action using.....");
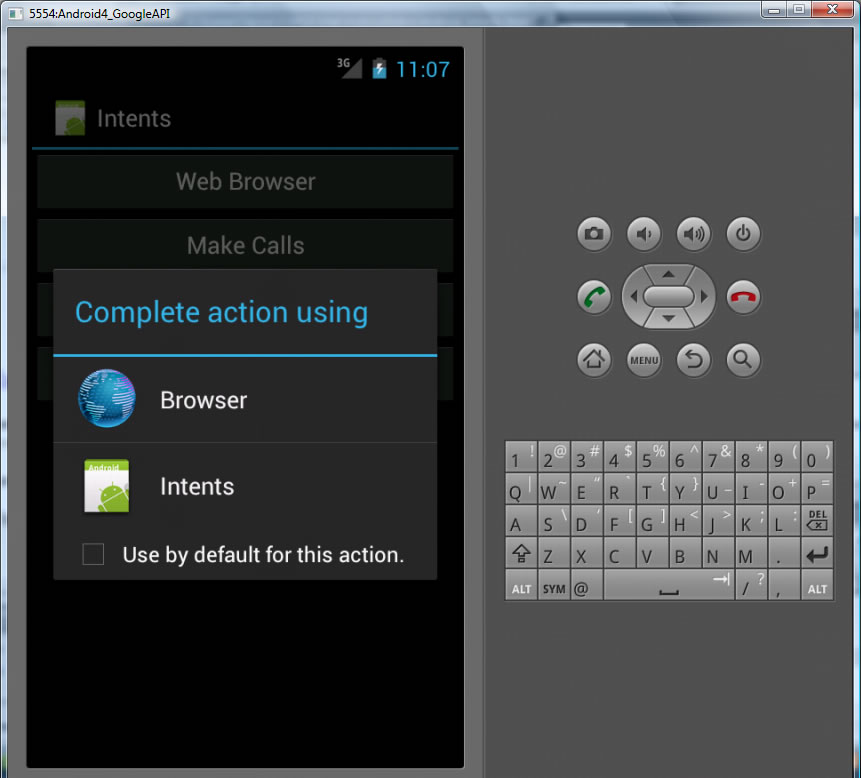
|