Activity
- presentation layer of an Android application, e.g. a screen which the user sees
- An Android application can have several activities and it can be switched between them during runtime of the application
- single, focused thing that the user can do
- takes care of creating a window for user
- presentation to the user
- full-screen windows
- floating windows
- embedding inside of another activity
TIP: Later we will learn about another kind of presentatation layer that are like "mini-activities" --called Fragements. You can create an activity made up of one or more Fragements --- a way of partitioning your screen space for more complicated GUIs (like frames in XHTML). Using multiple Fragements to create an Acitivity is useful particularly when designing for the larger real-estate of a tablet (over a phone) and can be used also effectively to alter the GUI between a phone (show only Activities with one fragment or a few in it) to a tablet (so more complex Acitivities combinging multiple Fragments)
void onCreate(Bundle savedInstanceState)
void onStart()
void onRestart()
void onResume()
void onPause()
void onStop()
void onDestroy()
- one time initialization stuff goes in onCreate
- stuff you need to setup each time the Activity is about to run goes in onResume
- resources like camera that you may use in Activity need to often be released in onPause and onStop methods and then "reactivated" in onResume method
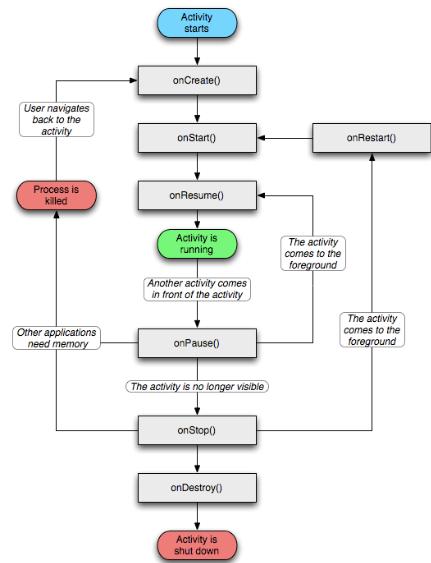
IMPORTANT: this will be more fully described with more detailed examples when we get to Intents.
(Up to now you have seen many examples where the Application has ONE Activity and it is started (launch) automatically when the Application is run....this is because it is declared in the AndroidManifest.xml file with the category <category android:name="android.intent.category.LAUNCHER"/> ) --- THIS IS WHY WE NEVER HAD TO Start our Activity
What we do when we want to start a different Activity (say ListActivity) from
|