Adroid Displaying Images using ImageView
SOURCE CODE
One way to display an image is to use an ImageView. Like many Android UI elements you can do it either in code/progamattically or you can specify it in part in the xml. Here is the example in Option 1 running
Option 1: Use xml <ImageView > tag inside an xml layout file 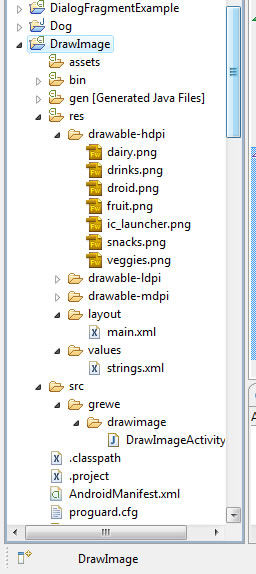
- Create Android Project named DrawImage with one Activity with the UI specified in main.xml that contains:
a TextView, ImageView and a Button
- See below main.xml that is in the res/layout folder.
- ADD IMAGES to project in any or all of the res/drawable-hdpi, drawable-mdpi, drawable-ldpi folders, the image is called droid.png (we have others we will use later)
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" > <TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal|center_vertical"
android:text="@string/hello" />
<ImageView
android:id="@+id/image_display"
android:src = "@drawable/droid"
android:layout_gravity="center_horizontal|center_vertical"
android:layout_width = "wrap_content"
android:layout_height ="wrap_content" /> <Button
android:id="@+id/btnChangeImage"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal|center_vertical"
android:text="Change Image" />
</LinearLayout>
(gets UI from the main.xml above)
import android.app.Activity;
import android.os.Bundle;
import android.widget.Button;
import android.view.View;
import android.widget.ImageView;
public class DrawImageActivity extends Activity {
//button in GUI defined in main.xml
Button image_button;
//ImageView object in GUI defined in main.xml
ImageView iview;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
//create handle to our Image View
iview = (ImageView) findViewById(R.id.image_display);
}
}
Altering Dynamically the Image that is Displayed
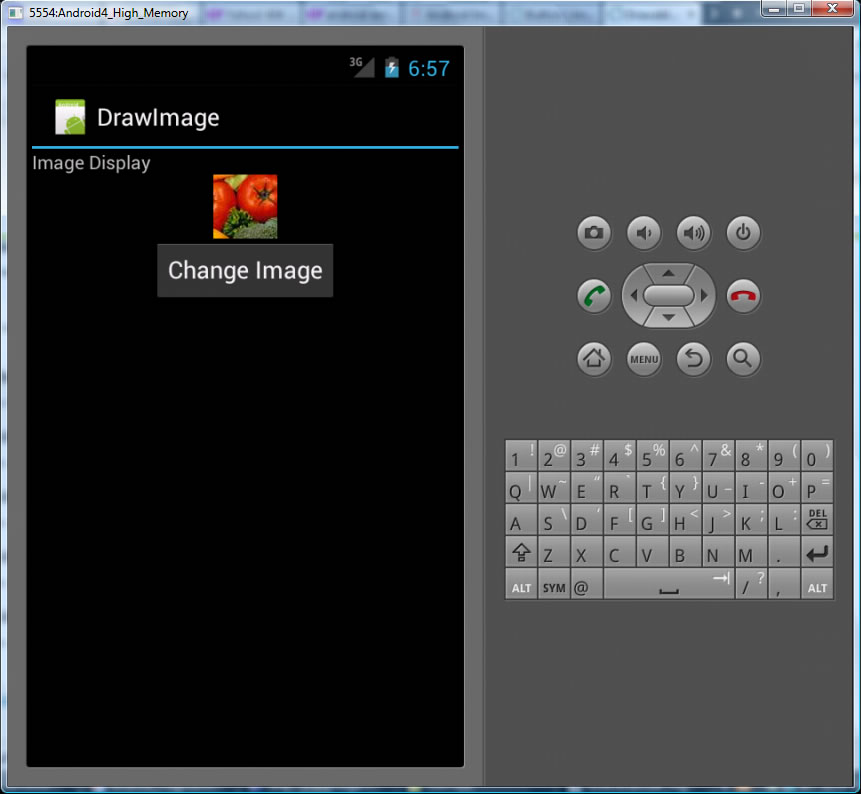 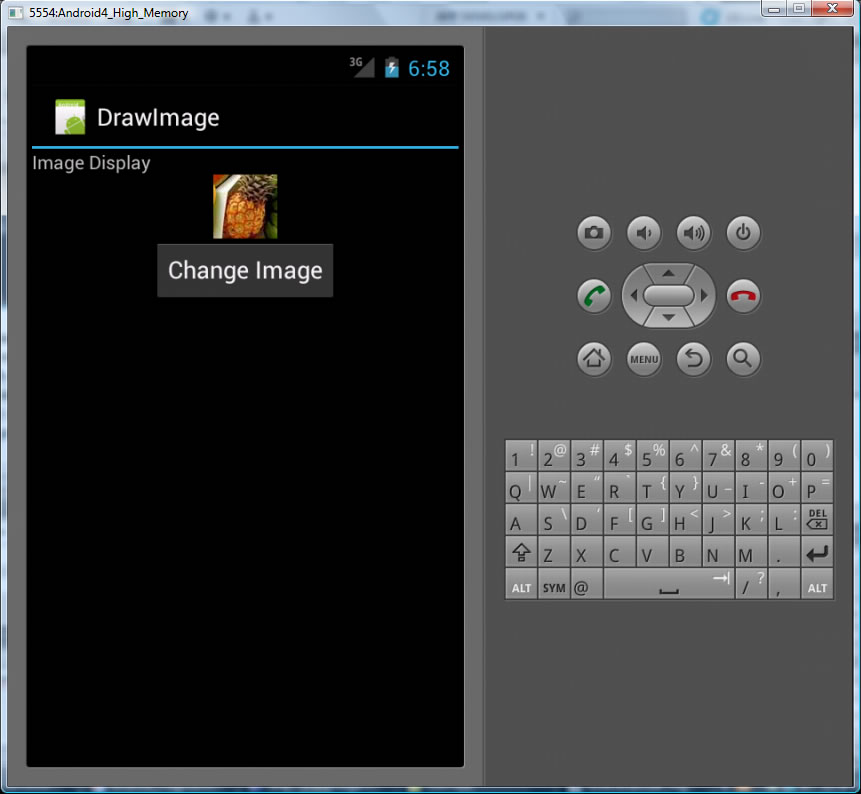
- NOTICE above in our res/drawable-hdpi folder we have multiple images. Lets write some code to now when the button is hit to cylce through the images of food we have stored there.
- we need to Alter DrawImageActivity.java in the following ways as reflected in the code below
- create a class variable representing the button in the interface called image_button
- create a class variable that is an array of resource ids representing our food images, called imageIDs[]
- create an index called image_index to loop through this array and restart to 0 when at the end of the array.
- add the onClick() eventlistener for this button to do our image display cycling through imageIDs array using image_index
(gets UI from the main.xml above)
import android.app.Activity;
import android.os.Bundle;
import android.widget.Button;
import android.view.View;
import android.widget.ImageView;
public class DrawImageActivity extends Activity {
//button in GUI defined in main.xml
Button image_button;
//ImageView object in GUI defined in main.xml
ImageView iview;
//Array of images that will cycle through and display in ImageView
// represented by their IDS
Integer[] imageIds = { R.drawable.veggies, R.drawable.fruit, R.drawable.dairy, R.drawable.snacks, R.drawable.drinks};
//image index to cycle through images defined in imageIds
int image_index =0;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
//create a handle to our button so we can do event handling on it
image_button = (Button) findViewById(R.id.btnChangeImage);
image_button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// change the image to next image in imageIds array
iview.setImageResource(imageIds[image_index]);
image_index++;
//if at end of image array return to the first image
if (image_index >= imageIds.length)
{ image_index =0; }
} });
//create handle to our Image View
iview = (ImageView) findViewById(R.id.image_display);
}
}
- invoke setImage with an integer R.drawable.* ---these are unique identifiers that can be used to represent files stored in the res/drawable-hdpi, mdpi and ldpi folders. The * = filename (without extension)
- How to invoke say from another fragment or Activity, maybe triggerd by some event-handing (see this exercise for a full example, look at ListFrag.java file):
//grab the container of the ImageView, here it is instance of DetailFrag associated with the
// Resource ID R.id.frag_capt (set in the detail_frag.xml used to create interface of DetailFrag)
DetailFrag frag = (DetailFrag) getFragmentManager().findFragmentById(R.id.frag_capt);
//make sure that the frag object is not null and is it part of current active view hierarchy
if (frag != null && frag.isInLayout()) { //change text and image
frag.setText(getCapt(item));
frag.setImage(R.id.newimageFilename); //where there is a file
//newimageFilename.png in res/drawable-hdpi folder
}
<ImageView> xml attributes --look at API for complete list!!!
ImageView class
¨Beyond the attributes of <ImageView>, the actual ImageView class has a rich(er) set of methods….see API…..
¨Here are some:
¨Here are some methods that can be used to set the ImageView contents
|