Java I/O
All of the I/O related classes is located in the java.io package. You should import this package at the top to use the classes.
Standard Stream Classes
(part of java.lang)
Standard Input Stream | System.in (of class InputStream)
|
---|---|
Standard Output Stream | System.out (of class PrintStream)
|
Standard Error Stream | System.err (of class PrintStream..will go to screen or special destination specified by host) |
Exercise 1
Basic Input and Output Stream Classes
(part of java.io)
public abstract class InputStream |
|
public abstract class Reader |
|
public abstract class OutputStream |
|
public abstract class Writer |
|
Derivative Input Stream Classes
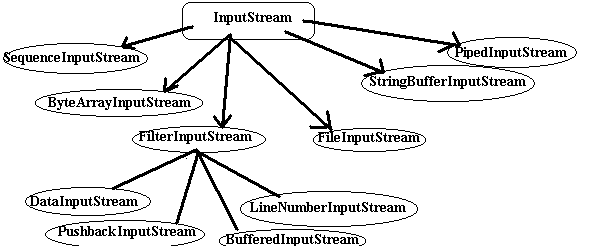
Opening/Closing Reading/Writing Files
(part of java.io)
- OPENING (for reading): FileInputStream
File file_handle = new File("Lynne.txt"); FileInputStream file_input = new FileInputStream(file_handle); OR FileInputStream file_input = new FileInputStream("Lynne.txt);
- OPENING (for reading): FileReader
File file_handle = new File("Lynne.txt"); FileReader file_input = new FileReader(file_handle); OR FileReader file_input = new FileReader("Lynne.txt);
- OPENING (for writing): FileOutputStream
File file_handle = new File("Lynne.txt"); FileOutputStream file_input = new FileOutputStream(file_handle); OR FileOutputStream file_input = new FileOutputStream("Lynne.txt);
- OPENING (for writing): FileWriter
File file_handle = new File("Lynne.txt"); FileWriter file_input = new FileWriter(file_handle); OR FileWriter file_input = new FileWriter("Lynne.txt);
- CLOSING: for any of the above classes
Stream.close();
- READING:DataInputStream is nice to use
- To convert any InputStream to a DataInputStream:
DataInputStream DIS = new DataInputStream( InputStream);
For example to convert standard input.DataInputStream DIS = new DataInputStream(System.in);
- DIS.readFloat() reads float from input stream
- DIS.readInt() reads int from input stream
- To convert any InputStream to a DataInputStream:
- READING:BufferedReader is nice to use
- Nice because will read in a line at a time from the input.
- To convert any Reader to a BufferedReader:
BufferedReader BR = new BufferedReader( Reader);
- BR.readLine() reads line from input and returns a String on which you can do further processing.
- WRITTING: DataOutputStream or BufferedWriter are nice to use (see example below)
Reading File Exercise |
Writing File Exercise |
Applet I/O
More exercises